How to debug Laravel App using Laravel Debugbar
Debugging Laravel applications can be a challenging task, especially when dealing with complex issues that are difficult to identify. However, with the help of Laravel Debugbar, you can simplify the debugging process and identify issues quickly and easily.
Here are the steps to debug a Laravel app using Laravel Debugbar:
1- Install Laravel Debugbar using composer:
composer require barryvdh/laravel-debugbar --dev
2- Once installed, Laravel Debugbar is enabled by default for local environments. You can access it by launching your application and opening the debug bar, which is typically located at the bottom of the page.
3- To enable Laravel Debugbar in production environments, you’ll need to update your .env file by setting the APP_DEBUG variable to true:
APP_DEBUG=true
4 – Laravel Debugbar provides various features to help you debug your application. Some of the useful features include:
- Timeline: Allows you to visualize the timeline of your application’s requests and identify slow-loading requests.
- Queries: Displays all the queries executed during a request and helps you identify any performance issues.
- Exceptions: Shows detailed information about any exceptions thrown during a request.
5- To use Laravel Debugbar effectively, you can place debugbar() helper function in your code, which allows you to debug your application at specific points in your code.
For example, you can place the following code in your controller to debug a variable:
public function index()
{
$data = ['name' => 'John', 'age' => 25];
debugbar()->info($data);
return view('index', $data);
}
This will display the contents of the $data variable in the Laravel Debugbar.
With these steps, you can easily debug your Laravel application using Laravel Debugbar and identify any issues in your code.
The APP_DEBUG
is true
will enable the debug bar. Update the APP_DEBUG
is false
to disable.
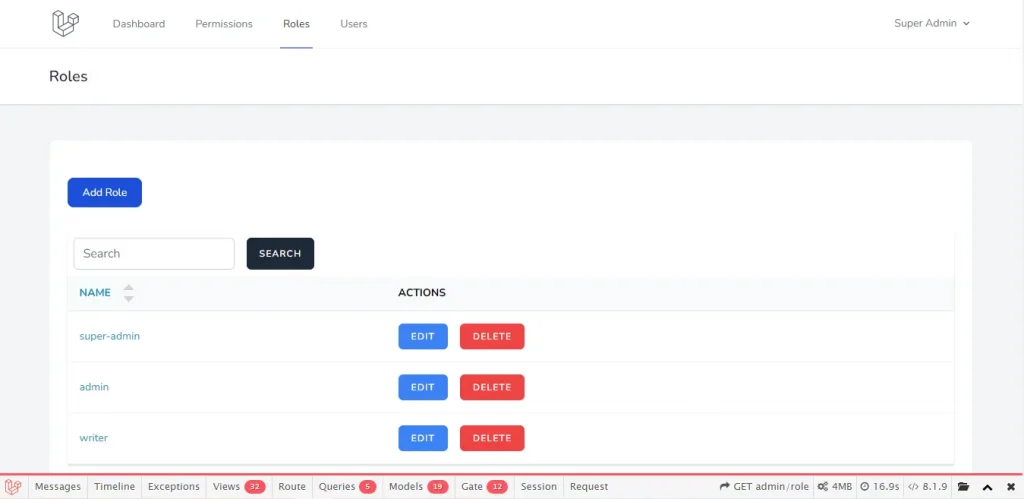
This is useful tab to analyze your query, because it will display the query statement and duration.
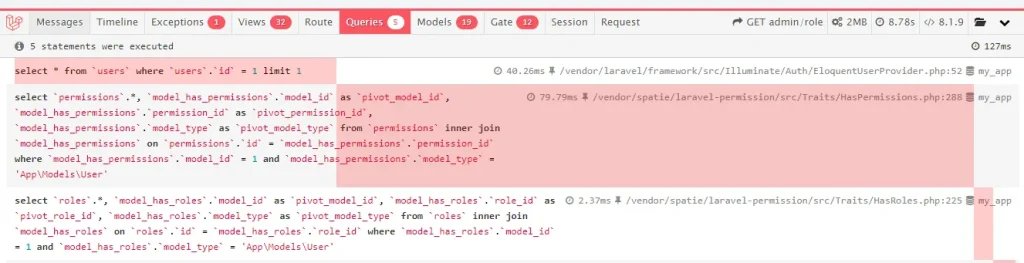