JavaScriptJquery
How to Calculate Age from Date of Birth in JavaScript
we are going to learn how we can calculate age from the given Date of Birth (DOB) in javascript to calculate the user’s age based on its DOB. To check user age eligibility and apply respective validation.
Here in this method, we add an input textbox and button tag. On button click, we call javascript function which gets the date from the input textbox and calculates age.
Here we going to add two textboxes i.e Date of Birth date and Current date. On button click
This is including part of code
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<form class="form-horizontal" action="">
<div class="form-group">
<label class="control-label col-sm-2" for="email">Date of Birth:</label>
<div class="col-sm-6">
<input type="text" class="form-control" id="dob" placeholder="dd/mm/yyyy" value="23/07/1994">
</div>
</div>
<div class="form-group">
<label class="control-label col-sm-2" for="pwd">Calculate Date:</label>
<div class="col-sm-6">
<input type="text" class="form-control" id="current_date" placeholder="dd/mm/yyyy" value="23/07/2022">
</div>
</div>
<div class="form-group">
<label class="control-label col-sm-2" for="pwd"> </label>
<div class="col-sm-6">
<div class="form-control" id="result_data"></div>
</div>
</div>
<div class="form-group">
<div class="col-sm-offset-2 col-sm-6">
<button type="button" class="btn btn-default" id="submit">Submit</button>
</div>
</div>
</form>
<script>
$(document).ready(function() {
$('#submit').click(function() {
var dob = $("#dob").val();
var current = $("#current_date").val();
var current = current.split('/');
var curdate = current[2] + '/' + current[1] + '/' + current[0];
var user_date = Date.parse(curdate);
var data_record = getAge(dob, user_date);
$("#result_data").text(data_record);
});
function getAge(mydob, today_date) {
var dob = mydob.split('/');
var curdate = dob[1] + '/' + dob[0] + '/' + dob[2];
var user_date = Date.parse(curdate);
var diff_date = today_date - user_date;
var diffY = Math.floor((diff_date) / (86400000 * 30.41 * 12));
var diffM = Math.floor((diff_date) / (86400000 * 30.41) - diffY * 12);
var diffD = Math.floor(((diff_date) / 86400000) - diffY * 12 * 30.41 - diffM * 30.41);
var finalY = Math.floor(diffY / 4);
if (finalY > 0) {
if (diffD > finalY) {
diffD = Math.ceil(diffD - finalY - 1);
} else {
if (diffM !== 0) {
diffM = (diffM - 1);
diffD = Math.ceil(31 - finalY);
} else {
}
}
}
return ("" + diffY + " Years " + diffM + " Months " + diffD + " Days");
}
});
</script>
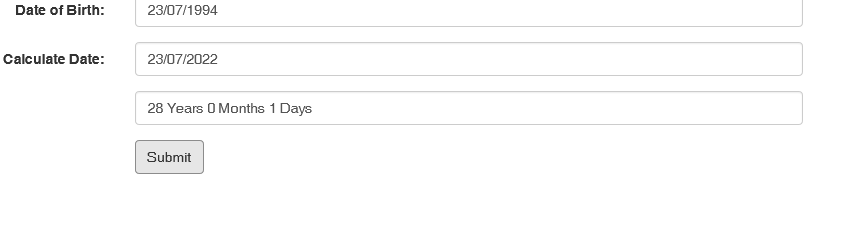